Wednesday, June 15, 2011
EL Expressions in Custom Tag Parameters
When recently working on a custom Java tag library, I had forgotten one twist. If you need your tag to have a parameter accept an EL expression, your TLD attribute entry needs to include <rtexprvalue>true</rtexprvalue>.
Also don't forget that the tag lib version should be at least 1.2 and the JSP version should be at least 2.0.
Also don't forget that the tag lib version should be at least 1.2 and the JSP version should be at least 2.0.
Friday, January 8, 2010
Shell script for file checking
I recently found I needed a simple way to determine if the correct number of daily files had been received and processed on my BPEL server. Since the server NFS mounts to a Windows share that has files being FTP'd to, there were a lot of places where a file might not make it. The shell script below checks for the number of instances of a file that has the date stamp in the file name and emails the count to me. This is set up on a daily (week days) cron.
#!/bin/bash
subject="Daily File Status"
email="name@domain.com"
eml_message=""
run_date=`date "+%Y%m%d"`
m1="*_"
m2="_*"
file_mask="$m1$run_date$m2"
count=0
#echo $file_mask
cd /BPEL/archive
for file in `ls -1 $file_mask`
do
# echo "Found file"
count=`expr $count + 1`
done
eml_message=`echo "Number of files found on the BPEL server for today was ( $count ). Normally this count should be (2)"`
echo $eml_message | /bin/mail -s "$subject" "$email"
A better solution is to check for the number of invoked instances...I'll be posting that next.
#!/bin/bash
subject="Daily File Status"
email="name@domain.com"
eml_message=""
run_date=`date "+%Y%m%d"`
m1="*_"
m2="_*"
file_mask="$m1$run_date$m2"
count=0
#echo $file_mask
cd /BPEL/archive
for file in `ls -1 $file_mask`
do
# echo "Found file"
count=`expr $count + 1`
done
eml_message=`echo "Number of files found on the BPEL server for today was ( $count ). Normally this count should be (2)"`
echo $eml_message | /bin/mail -s "$subject" "$email"
A better solution is to check for the number of invoked instances...I'll be posting that next.
Thursday, September 10, 2009
My Review of MBT Men's Tariki - Walnut Oiled Leather - On Sale!
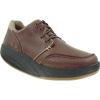
Free shipping and free return shipping on all MBT Footwear.
Each pair of MBTs include an instructional DVD instruction card.
What...
Best work shoes I've had
Development geek Sanford, FL 9/10/2009
4 5
Sizing: Feels true to size
Width: Feels too narrow
Pros: Stable, Looks Great, Comfortable, Durable
Cons: Width a tad small
Best Uses: Business Casual, Casual Wear
Describe Yourself: Comfort Driven
These are great. Comfort is comparable to Clark's mens shoes. They look great for a casual office environment, so these are now my daily wear for work.
Initially the uppers are quite stiff, since they are leather, so you'll want to break them in slowly.
The width is a tad narrow, but I expect as they get more wear on them this will cease to be an issue.
Tuesday, April 28, 2009
Tuesday, December 30, 2008
How to Access BPEL Variables in a Java Embed Activity
In your embedded Java you can use:
javaVar = getVariableData("BPEL_Variable_Name");
and
setVariableData("BPEL_Variable_Name", javaVar);
Obviously, the types need to match up!
Also useful for debugging/logging purposes is:
addAuditTrailEntry("My Message Goes Here:",optionalJavaVarValueToLog);
javaVar = getVariableData("BPEL_Variable_Name");
and
setVariableData("BPEL_Variable_Name", javaVar);
Obviously, the types need to match up!
Also useful for debugging/logging purposes is:
addAuditTrailEntry("My Message Goes Here:",optionalJavaVarValueToLog);
Wednesday, October 29, 2008
How to Create A Multiple Entry Point BPEL Process Using Pick
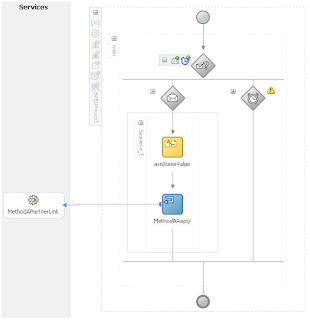
In RIA development we want frequently want to consume web services. From a organizational point of view we typically want related methods to be provided by the same provider. If the web service is being provided via a BPEL process, how can we do that?
The answer is to use a BPEL process with a Pick activity.
Steps:
1) Create a normal synchronous BPEL process. Let the wizard create it's default input and output variables, as these won't be used.
2) Drop your custom input and output XSD files into the
3) Delete the the client partner link. When you do you'll be asked if you want to delete the Partner link -- answer Yes. You'll also be asked if you want to delete the WSDL file -- answer No to this.
4) Drop a Pick activity between the existing Receive and Reply activities. Now delete the Receive and Reply activities.
5) Edit the project WSDL file:
a) In the types section, delete the imports for the default XSD file, and add imports for your custom XSD files
b) Delete the existing messages and create a message for each request and response message you will be defining
c) Delete the existing portType definition. Add a new one, and under the portType add a new operation for each method you are implementing in this BPEL process via the Pick
d) Change the existing partnerLinkType to use your new portType
6) You will need to create an onMessage branch in the Pick for each operation being implemented:
a) Double click the message icon and select the partner link to associate with this message. On the first message you will need to create a new partner link.
b) Create the new input message variable, selecting the desired message type.
c) Select the appropriate operation type. This will bind the partner link message to this message branch.
7) You will need to add a sequence (or a scope/sequence combination) to each message branch and provide the rest of the activities within it.
8) If you are returning multiple return types (most likely), each branch will need a Reply activity at then end of it to return the output to the client.
Monday, October 27, 2008
Enable/Unlock OID User Account Using Java API
How to programmatically enable and/or unlock a user's OID account using the Java API? Let's take a step by step look:
First get a reference to the directory context:
ctx = oracle.ldap.util.jndi.ConnectionUtil.getDefaultDirCtx(ldapHost, ldapPort, ldapUserCN, ldapCred );
ldapUserCN will most likely be something like "cn=orcladmin" and ldapCred will be the password for that CN.
Next, we build an attribute set that we are going to update. This will do the account enable:
attrSet = new javax.naming.directory.BasicAttributes();
attrSet.put("orclIsEnabled", "ENABLED");
ctx.modifyAttributes(userDN, javax.naming.directory.DirContext.REPLACE_ATTRIBUTE, attrSet);
userDN here will be the full DN of the user we are modifying. We can also force a new password by putting the attribute "userpassword" in the attribute set and giving it the new password as its value.
Finally, we will do the unlock:
attrSet = new javax.naming.directory.BasicAttributes();
attrSet.put("orclpwdaccountunlock","1");
ctx.modifyAttributes(userDN, javax.naming.directory.DirContext.ADD_ATTRIBUTE, attrSet);
You should note that if you are modifying the user password programmatically, the password must adhere to all of the normal password rules set up for your OID instance.
The code can throw javax.naming.directory.InvalidAttributeValueException , which can be caused by a password not adhering to the OID policies.
First get a reference to the directory context:
ctx = oracle.ldap.util.jndi.ConnectionUtil.getDefaultDirCtx(ldapHost, ldapPort, ldapUserCN, ldapCred );
ldapUserCN will most likely be something like "cn=orcladmin" and ldapCred will be the password for that CN.
Next, we build an attribute set that we are going to update. This will do the account enable:
attrSet = new javax.naming.directory.BasicAttributes();
attrSet.put("orclIsEnabled", "ENABLED");
ctx.modifyAttributes(userDN, javax.naming.directory.DirContext.REPLACE_ATTRIBUTE, attrSet);
userDN here will be the full DN of the user we are modifying. We can also force a new password by putting the attribute "userpassword" in the attribute set and giving it the new password as its value.
Finally, we will do the unlock:
attrSet = new javax.naming.directory.BasicAttributes();
attrSet.put("orclpwdaccountunlock","1");
ctx.modifyAttributes(userDN, javax.naming.directory.DirContext.ADD_ATTRIBUTE, attrSet);
You should note that if you are modifying the user password programmatically, the password must adhere to all of the normal password rules set up for your OID instance.
The code can throw javax.naming.directory.InvalidAttributeValueException , which can be caused by a password not adhering to the OID policies.
Subscribe to:
Posts (Atom)